Here is the implemented code in c (-std=c99).
#include <stdio.h>
#include <stdlib.h>
#define M 5
#define N 4
int M_data[M] = {1,2,3,4,5};
int N_data[N] = {6,7,8,9};
typedef struct node {
int data;
struct node * next;
}node;
void join(node *m,node *n) {
node * p = n;
while(p->next != NULL) p = p->next;
p->next = m;
}
node * bulk_insert(int list_data[],int size) {
node * head = NULL;
for(int i=0;i<size;i++) {
node * newnode = malloc(sizeof(node));
newnode->data = list_data[i];
newnode->next = NULL;
if(head == NULL) {
head = newnode;
}else {
node * temp = head;
while(temp->next != NULL) temp = temp->next;
temp->next = newnode;
}
}
return head;
}
void display(node *);
void list_dealloc(node *); /*deallocation prototype*/
int main() {
node * m = NULL;
node * n = NULL;
// insert m_list data
m = bulk_insert(M_data,M);
n = bulk_insert(N_data,N); // commenting this causes runtime error
// is list n is empty
printf("\n before join operation :\n");
display(m);
display(n);
join(m,n);
printf("\n after join operation :\n");
display(m);
display(n);
//list_dealloc(m); no need now
list_dealloc(n); // OK
return 0;
}
void display(node *head) {
while(head != NULL) {
printf("%d->",head->data);
head = head->next;
}
printf("null\n");
}
void list_dealloc(node * head) {
while(head != NULL) {
node * temp = head;
head = head->next;
free(temp);
}
}
With both n and m and n being non-empty linked list, then,
O/P:
before join operation :
1->2->3->4->5->null
6->7->8->9->null
after join operation :
1->2->3->4->5->null
6->7->8->9->1->2->3->4->5->null
With n being empty linked list, then,
O/P:
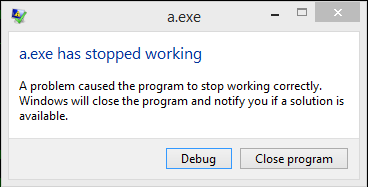
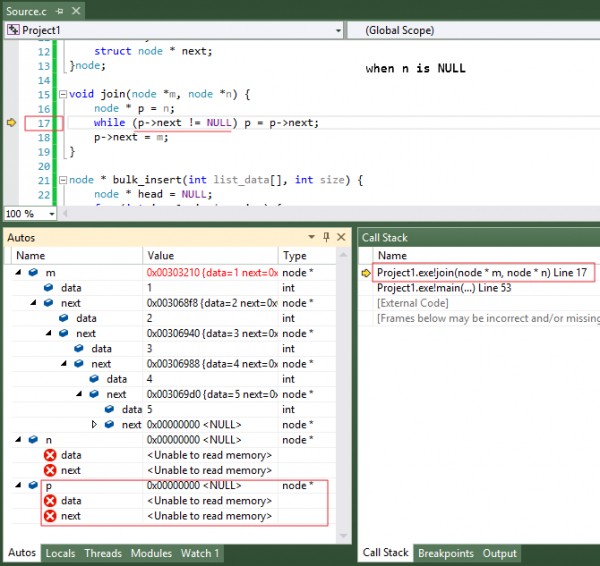
Correct Answer: $B$